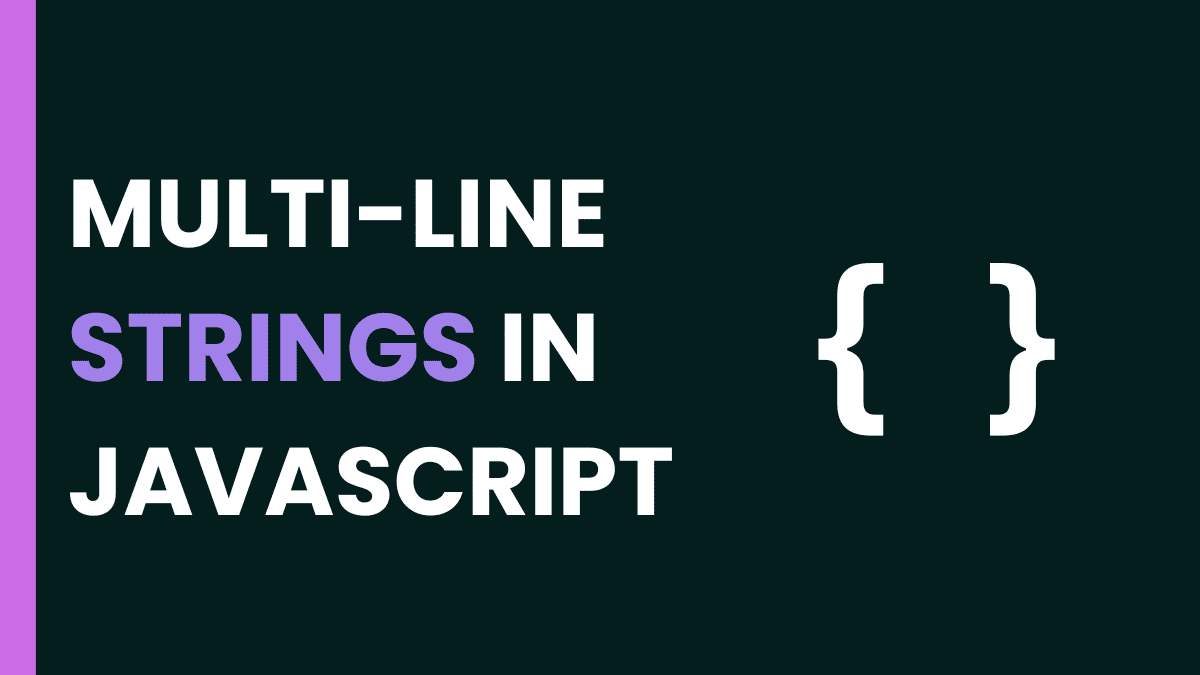
JavaScript, HTML, and CSS are the three key technologies and foundations of Web development and most of the web pages.
JavaScript is a dynamic programming language that brings web pages to life by adding interactivity and functionality. It’s the magic behind the scenes that makes web pages more than just static text and images.
HTML (HyperText Markup Language), on the other hand, is the skeleton of the web. Big ups to Tim Bernes-Lee (and if you don’t know now you know …) It provides the structure and content of a webpage, from paragraphs to headers, links to images, and everything in between. CSS (Cascading Style Sheets) is the stylist of the web. It describes how HTML elements should be displayed, controlling layout, colors, fonts, and more. It’s what makes our websites look good.
Now, when it comes to integrating JavaScript multiline strings with HTML and CSS, it’s a game-changer. Multiline strings in JavaScript allow us to write long strings in a more readable format without concatenation or worrying about white space. This integration can make your code cleaner, easier to read, and maintain. It’s an essential skill for any aspiring web developer and a powerful tool for seasoned professionals.
In this article, we will delve deeper into how to integrate JavaScript multiline strings with HTML and CSS effectively. Stay tuned!
JavaScript Multiline Strings
Multiline strings in JavaScript let you write text that goes over more than one line in your code. Usually, JavaScript uses strings that are just one line long and are surrounded by single or double quotes.
But, when you have a lot of text, it can make your code hard to read and tricky to keep neat. That’s where multiline strings come in handy. They make your code easier to read and keep tidy, especially when you’re working with big chunks of text, templates, or queries.
In other words, a JavaScript multiline string is a string that spans multiple lines. This makes it simpler for developers to read the code and make changes to it. There are several ways to create multiline strings in JavaScript:
- Template Literals: Introduced in ECMAScript 6 (ES6), template literals are denoted by backticks (`). They can be used to create multiline strings simplyTemplate literals also support variable interpolation.
- Backslashes: You can use backslashes (\) to make multiline strings in your code. But when you print the string, it still shows up on one line.
- Newline Character: You can use the newline character (\n) to make strings that print on multiple lines. But this way is harder than using template literals.
- String Concatenation: You can use the plus sign (+) to join strings together over several lines. If you want each piece to print on a different line, you can add a new line character at the end of each piece.
If you want a modern and flexible way to make multiline strings, template literals are the best choice. But if you need to ensure your code works in all browsers, you might need to use a different way.
Benefits of using JavaScript multiline strings with HTML and CSS
JavaScript multiline strings let you write text that goes over more than one line. You need to use them with HTML and CSS for many reasons:
- Enhanced Readability: Multiline strings make the code easier to read, especially when you have a lot of text, templates, or queries. This helps developers to change and improve the code.
- Better Maintainability: Multiline strings keep the code tidy without breaking the string. This is good when you have a lot of text data.
- Efficiency: Multiline strings help you make strings that go over many lines in a better way. This can be helpful when you have a lot of text or when you want to show the code nicely.
- Functionality: Multiline strings are important for template literals and regular expressions. They let you put expressions inside the string that will be calculated and added to the string.
- Compatibility: With the new version of JavaScript (ES6), you can make multiline strings easily by using backticks. This works well in most browsers. Using JavaScript multiline strings with HTML and CSS makes your code look better and easier to read. It also lets you do more things with your web pages.
Integrating JavaScript multiline strings with HTML and CSS
Integrating JavaScript multiline strings with HTML and CSS involves a few steps. First, you create a multiline string in JavaScript using backticks (`) to form a template literal. This allows you to write a string that spans multiple lines, improving the readability of your code.
Next, you embed your JavaScript code within your HTML file. This is done by enclosing your JavaScript code in <script> tags. This allows your JavaScript code, including your multiline string, to interact with your HTML content.
To display your multiline string on your webpage, you use JavaScript to modify the HTML content. This can be done using various JavaScript methods that interact with the HTML Document Object Model (DOM), such as document.getElementById() or document.querySelector().
Finally, you can style your multiline string using CSS. This is done by adding a <style> tag to your HTML file or linking to an external CSS file. Within your CSS, you can specify styles for the HTML elements that are displaying your multiline string.
Now let’s see it in action:
Process: JavaScript Multiline Strings with HTML and CSS
HTML Structure
Let’s create a basic structure by keeping the fact in mind that we will add Javascript and CSS codes in upcoming steps.
<html>Â
<head>
<title> Create multi-line strings </title>
</head>
<body>
<div class="container">
<h1>Â JavaScript Multiline Strings with HTML and CSS </h1>
<p> Multi-line string creation example using JavaScript with HTML and CSS </p>
<p> Click on the button to see output of JavaScript Multiline Strings</p>
<button onclick="showMultilineString()"> Display Multiline </button>
<div id="multiline"> </div>
</div>
</body>
</html>
Javascript Multiline
<script>
function showMultilineString() {
 multilineString =
"<div>" +
 "<h3>This is an example of JavaScript Multiline Strings with HTML and CSS</h3>" +
 "</br><p> EyeUniversal’s commitment to innovation and transparency has kept us growing for more than 15 years." +
 "Whether your project involves design, development, or marketing," +
 "We deliver our work On time. On budget. From proven professionals.</p> " + "</div>" ; document.getElementById('multiline').innerHTML = multilineString;Â
     }Â
</script>
</div>
Now time for styling using CSS
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
background-color: #f0f0f0;
}
.container {
max-width: 800px;
margin: 0 auto;
padding: 20px;
}
h1 {
color: #4CAF50;
text-align: center;
}
p {
color: #333;
line-height: 1.6;
text-align: center;
}
button {
display: block;
width: 200px;
height: 50px;
margin: 20px auto;
background-color: #4CAF50;
color: #fff;
border: none;
border-radius: 5px;
cursor: pointer;
}
button:hover {
background-color: #45a049;
}
#multiline {
background-color: #ddd;
border-radius: 5px;
padding: 20px;
margin-top: 20px;
}
Output
Now it is time for seeing codes in action. https://codepen.io/skyuvraj/pen/NWJGzZx
Before click:

After Click:

This is just one basic example, it can be used in many cases and many types of tasks. Check the use cases below.
Use cases
JavaScript multiline strings let you write text that spans more than one line. You can use them with HTML for different purposes:
- HTML Templates: You can make HTML templates in JavaScript with multiline strings. They help you see the shape and make changes. For example, you can use multiline strings to make an HTML table or form that changes with the data.
- Dynamic Content Generation: You can use multiline strings to make or change HTML content on the fly. This is good for web pages that change without reloading.
- Embedding Scripts: You can use multiline strings to put scripts inside HTML. This can be helpful when you want to add JavaScript to your HTML.
- SQL Queries: In JavaScript apps that work with databases, you can use multiline strings to make SQL queries. This makes it easier to read and edit the queries in the code.
Remember, JavaScript multiline strings can be very helpful, but you should use them wisely and make sure they make your code easier to read and maintain.
Some Drawbacks You Should Know About
Integrating JavaScript multiline strings with HTML and CSS can have a few drawbacks:
- Inconsistent Browser Support: While most modern browsers support ES6 features like template literals for multiline strings, some older browsers do not. This could lead to compatibility issues.
- Code Complexity: Using multiline strings can sometimes make the code more complex, especially when dealing with large blocks of text. This could potentially make your code harder to read and maintain.
- Risk of Errors: If you’re not careful, it’s easy to introduce errors when using multiline strings. For example, forgetting to properly escape special characters or not matching the types of quotes can lead to syntax errors.
- Potential for Cross-Site Scripting (XSS) Attacks: If you’re inserting user input into your webpage using methods like innerHTML, there’s a risk of XSS attacks if the input is not properly sanitized. This is a serious security concern and needs to be handled carefully.
- Performance Impact: If you’re using multiline strings to generate large amounts of HTML dynamically, it could potentially have a performance impact on your webpage. This is especially true if you’re repeatedly updating the DOM.
While JavaScript multiline strings can be handy, it’s important to use them appropriately and be aware of the potential drawbacks.
Conclusion
JavaScript multiline strings let you write text that goes over more than one line. You don’t need to use special characters or join the lines. They make your code easier to read and change, especially when you have a lot of text, templates, queries, or scripts.
You can use JavaScript multiline strings with HTML and CSS by using backticks and template literals. However, you should also be aware of some known problems of using JavaScript multiline strings, like browser support, speed, and security. Hence, you need to use them carefully and correctly, and always check your code before using it.