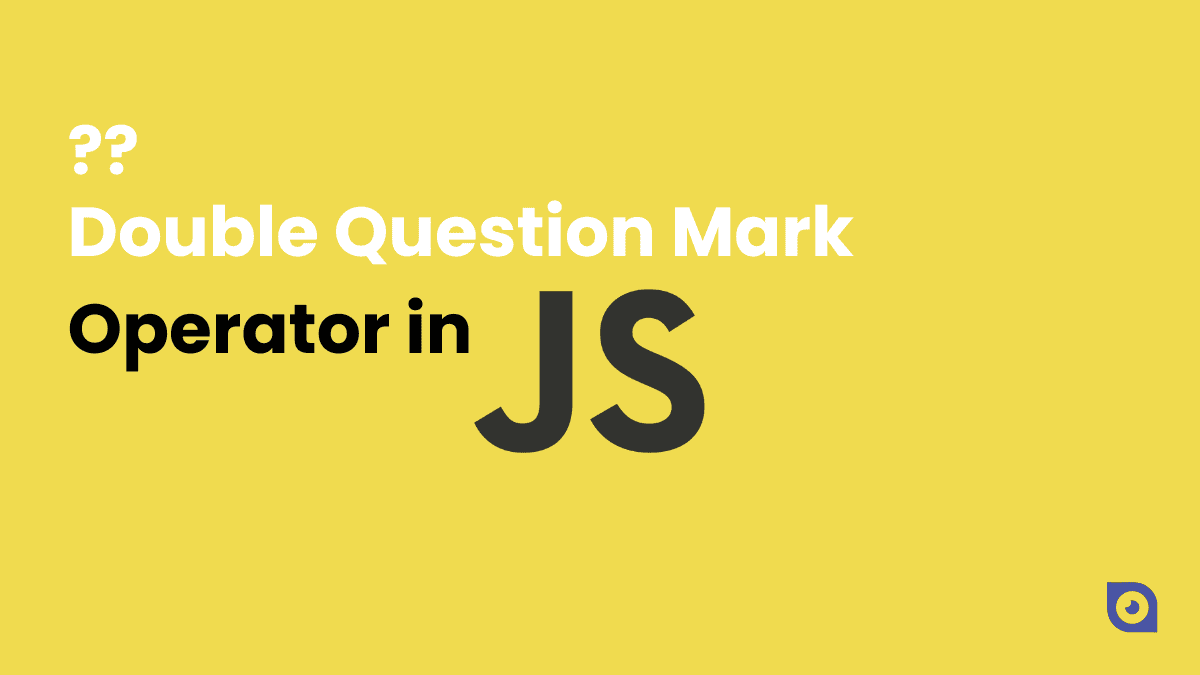
JavaScript, a high-level, interpreted programming language, is a core technology of the World Wide Web. It’s primarily used to enhance web pages to provide a more user-friendly experience. JavaScript supports portions of object-oriented programming (loosely based from OG Java syntax) and allows for interactive effects within a specific environment.
In JavaScript, operators are symbols that are used to perform operations on operands. Operators can manipulate individual items and variables, and they play a vital role in writing scripts. Examples of operators in JavaScript include arithmetic operators such as +, -, *, and /, assignment operators like =, comparison operators like == and !=, logical operators like && and ||, and many more.
Double Question Mark Operator was introduced in ECMAScript 2020. It is the Nullish Coalescing Operator (??). Now to understand the Double Question Mark Operator properly, you need to understand what is Null value first and that’s exactly where we are heading to.
In this article, we’ll first discuss the Null value in Javascript and then we will dive deeper into the JavaScript Double Question Mark Operator or Nullish Coalescing Operator, its uses, advantages, and potential pitfalls. Whether you’re a beginner just starting with JavaScript or an experienced developer looking to brush up on the latest features, this article aims to provide a comprehensive understanding of the ?? operator.
Stay tuned for more insights into this fascinating aspect of JavaScript ?? operator!
Understanding Null and Undefined in JavaScript
In JavaScript, null and undefined are two distinct types that each represent the absence of a value. However, they are used in slightly different scenarios and have different implications.
What is Null?
In JavaScript, null is a special value that represents no value or no object. It is intentional, and explicit absence of any object value. For example, when you want to indicate that a variable should have no value, you can assign it the null value.
let data = null;
console.log(data); // Outputs: null
What is Undefined?
Undefined in JavaScript means a variable has been declared, but has not yet been assigned a value. If you try to log an uninitialized variable, JavaScript returns undefined.
let data;
console.log(data); // Outputs: undefined
Differences Between Null and Undefined
While both null and undefined signify the absence of a value, they are used in different contexts:
- Explicit vs Implicit: null is used when you want to explicitly indicate the absence of a value. On the other hand, undefined is returned by JavaScript when a variable has been declared, but not initialized.
- Typeof Operator: The typeof operator in JavaScript returns “object” for null and “undefined” for undefined.
console.log(typeof null); // Outputs: "object"
console.log(typeof undefined); // Outputs: "undefined"
- Comparisons: null and undefined are loosely equal (==), but not strictly equal (===).
console.log(null == undefined); // Outputs: true
console.log(null === undefined); // Outputs: false
Understanding the difference between null and undefined is crucial as it can help prevent bugs and make your code more readable and maintainable.
Common Problems Encountered Due to Null or Undefined
In JavaScript, variables can often have the value null or undefined. This usually happens when a variable is declared but not assigned a value, or when an object property or array element that does not exist is accessed. These null or undefined values can lead to unexpected behavior and bugs in the code.
For example, consider the following code snippet:
let user = {};
console.log(user.name.length); // TypeError: Cannot read property 'length' of undefined
In this case, since user.name is undefined, trying to access its length property results in a TypeError.
How These Problems Affect Code Execution?
Such errors not only cause the current operation to fail but also halt the entire script execution. This can lead to a poor user experience, especially if the error is not handled properly.
Moreover, in JavaScript, null and undefined are considered falsy values. However, other falsy values include false, 0, NaN, ” (empty string), and -0. This can lead to unexpected results when using logical operators for default value assignment.
let count = 0;
let total = count || 10; // total is now 10, not 0
In this case, we might expect the total to be 0 (the value of count), but because 0 is a falsy value, the || operator instead assigns the value 10 to the total.
The Double Question Mark Operator to the Rescue
This is where the Nullish Coalescing Operator (??) comes into play. The ?? operator returns the first operand if it is not null or undefined. Otherwise, it returns the second operand.
let count = 0;
let total = count ?? 10; // total is now 0, as expected
In this case, even though 0 is a falsy value, the ?? operator correctly assigns the value 0 to the total, because 0 is not null or undefined. Let me explain this JavaScript Double Question Mark Operator to you in detail.
What is JavaScript Double Question Mark Operator?
The Nullish Coalescing Operator, also known as the Double Question Mark Operator (??), is a logical operator that returns its right-hand side operand when its left-hand side operand is null or undefined and otherwise returns its left-hand side operand.
This operator returns the first argument if it’s not null or undefined. Otherwise, it returns the second argument. While it might seem similar to the logical OR (||) operator, there’s a key difference: the ?? operator only returns the second argument if the first one is null or undefined, not if it’s an empty string, the number 0, or boolean false.
In simple words, we can say that the Nullish Coalescing Operator (??) is a way of choosing a value from two options when you don’t know if one of them is missing or not. For example, suppose you have a variable called name that may or may not have a value assigned to it. You want to use this variable to greet someone, but if it is missing, you want to use a default value like “Guest”. You can write this using the ?? operator like this:
let name; // this variable may or may not have a value
let greeting = "Hello, " + (name ?? "Guest"); // use the ?? operator to choose a value
console.log(greeting); // this will print "Hello, Guest" if name is null or undefined, otherwise it will print "Hello, " followed by the value of name
The ?? operator is different from the logical OR (||) operator, which also chooses a value from two options, but based on whether they are true or false. A value is truthy if it is not one of these: false, 0, “”, null, undefined, or NaN. A value is falsy if it is one of these.
For example, suppose you have a variable called score that may or may not have a value assigned to it.
You want to use this variable to display a message, but if it is missing, you want to use a default value like 0. You can write this using the || operator like this:
let score; // this variable may or may not have a value
let message = "Your score is " + (score || 0); // use the || operator to choose a value
console.log(message); // this will print "Your score is 0" If the score is falsy, otherwise it will print "Your score is " followed by the value of the score
However, if you use the ?? operator instead of the || operator, you will get a different result. For example, suppose the value of the score is 0. Then, using the ?? operator, you will get this:
let score = 0; // this variable has a value of 0
let message = "Your score is " + (score ?? 100); // use the ?? operator to choose a value
console.log(message); // this will print "Your score is 0" because the score is not null or undefined
But using the || operator, you will get this:
let score = 0; // this variable has a value of 0
let message = "Your score is " + (score || 100); // use the || operator to choose a value
console.log(message); // this will print "Your score is 100" because the score is falsy
As you can see, the ?? operator only considers null and undefined as missing values, while the || operator considers any false value as missing. This can make a difference when you want to use a value that is false but not missing, such as 0, “”, or false.
The Nullish Coalescing Operator (??) thus solves a major problem for developers by providing a reliable way to set default values and avoid TypeErrors caused by null or undefined values. It leads to cleaner, more predictable code, and a better JavaScript development experience.
I hope this explanation and example helped you understand the Nullish Coalescing Operator better.
Potential Pitfalls and How to Avoid Them
While the Nullish Coalescing Operator (??) is a powerful tool in JavaScript, it’s not without its potential pitfalls. Here are some common mistakes and best practices to avoid them:
Common Mistakes
One common mistake is misunderstanding the difference between null, undefined, and other “falsy” values in JavaScript. The ?? operator only checks for null and undefined, not other falsy values like 0, false, NaN, or ”. This can lead to unexpected results if you’re not careful.
let count = 0;
let total = count ?? 10; // total will be 0, not 10
In the above example, the total will be 0, not 10, because 0 is not considered a null value.
Best Practices
To avoid these pitfalls, here are some best practices:
- Understand the difference between nullish and falsy values: Before using the ?? operator, make sure you understand the difference between nullish (null and undefined) and other falsy values (0, false, NaN, ”, etc.).
- Use ?? for default values: The ?? operator is best used for providing default values for variables that might be null or undefined.
- Combine ?? with Optional Chaining (?.) for deep property access: When accessing properties deep within an object structure, consider using the ?? operator in combination with Optional Chaining (?.) to avoid TypeError.
let user = {
profile: {
name: 'John Doe',
age: null
}
};
let age = user?.profile?.age ?? 18; // age will be 18
In the above example, the age will be 18, because the user.profile.age is null.
By understanding these potential pitfalls and following these best practices, you can use the Nullish Coalescing Operator (??) effectively and safely in your JavaScript code.
Conclusion
Throughout this article, we’ve taken a deep dive into the intricacies of JavaScript Double Question Mark Operator (??). We’ve explored the nuances of null and undefined, the common problems they can cause, and how the ?? operator can help mitigate these issues.
We’ve also discussed the potential pitfalls of using the ?? operator and provided best practices to avoid them. By understanding these concepts, you can write more robust and efficient JavaScript code.
The Nullish Coalescing Operator (??) is a powerful tool in your JavaScript toolkit. It’s not just about handling null and undefined – it’s about writing cleaner, more readable code. It’s about making your code more predictable and less prone to bugs.
So, why wait? Start experimenting with the Nullish Coalescing Operator (??) in your code today. Embrace the power and simplicity it brings to your JavaScript development. Keep coding!